I have to start somewhere so I started wtih super baby steps. Downloading the .NET Core 2 nightly build and trying to create a simple console app. Right away I failed miserably. The reason? The nightly builds have already jumped the shark! They are working on 2.1.0 and I accidentally grabbed that. And that was a little too bleeding edge for me.
There is a docker image that you can use quite easily but I wanted to try CLI, VS Code and Visual Studio. Therefore I wanted to just install the bits right on my machine.
What I’ll show you are my first tests I did on macOS and on Windows 10. I like to do this just to make sure things are actually running properly. Note that on macOS, I just installed this new version directly on my machine where I have other versions of .NET Core. Key is that there is no production work on there dependent on other versions, so I won’t mess up anything important. The versions can live side by side. It’s just that for someone like me who “knows enough to be dangerous”, it’s easy to get tangled up with the versions even though I know tricks like creating a local nuget.config file and also specifying a version in global.json. On Windows, however, I am using a clean VM that has no other versions of .NET on it. That’s the smart way anyway. Although I did try the not smart way of installig it on my machine that already has all kinds of versions of all kinds of frameworks on it and even with my versioning tricks, I could not get 2.0.0 to do a restore or build.
I mentioned above that I originally downloaded the wrong version of the SDK. (Note that the typical SDK install also includes the runtime….so I got the wrong versoins of both. You can read the gory details of that in this GitHub issue which I kept updating as I sorted the problem out.) I want to stick with .NET Core 2.0.0. The installer for that is tucked away in a branch of github.com/dotnet/cli rather than grabbing the absolute latest from the master. Instead go to https://github.com/dotnet/cli/tree/release/2.0.0. There is a solid 2.0.0 version — 2.0.0-preview2-006391. That’s the one I’m using on Windows and macOS.
Make Sure NuGet Knows Where to Find Packages
You can update your global NuGet.Config before creating projects. Alternatively, you can create a project and then add a local NuGet.config file to it.
On Mac, the global config file at [user]/.nuget/NuGet. Here s a screenshot if like me, macOS is not your primary platform and you still struggle with these things.
In Windows, it’s at %APPDATA%\NuGet\.
I keep a link to the “Configuring NuGet behavior” doc handy for when I forget where to find that.
I added these two keys to my PackageSources section:
<add key="nuget.org" value="https://api.nuget.org/v3/index.json" protocolVersion="3" /> <add key="dotnet-core" value="https://dotnet.myget.org/F/dotnet-core/api/v3/index.json"/>
Now it’s time to create a project
I’m on the Mac, so, to Terminal we will go.
I created one folder for each inside the EFCore20 folder:
netcore2console
netstandard2lib
Then I cd’d into the netcore2console app to create that app with the command:
<?xml version="1.0" encoding="utf-8"?> <configuration> <packageSources> <addkey="nuget.org"value="https://api.nuget.org/v3/index.json"protocolVersion="3"/> <addkey="dotnet-core"value="https://dotnet.myget.org/F/dotnet-core/api/v3/index.json"/> </packageSources> </configuration>
dotnet restore
dotnet build
dotnet run
Hello World!
code .
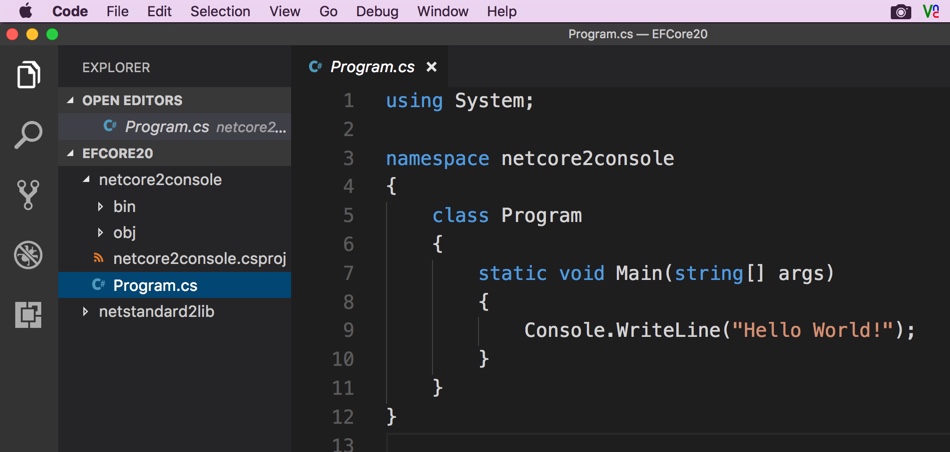
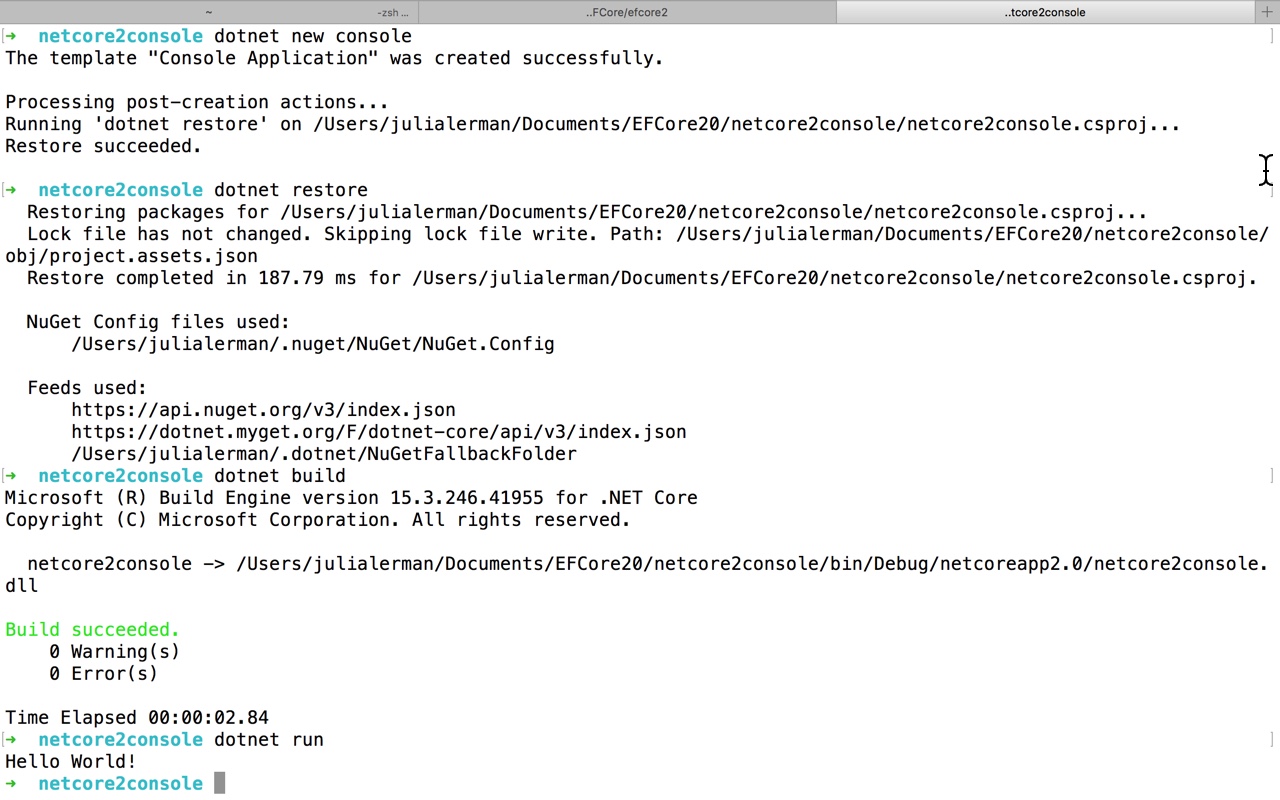
dotnet new library
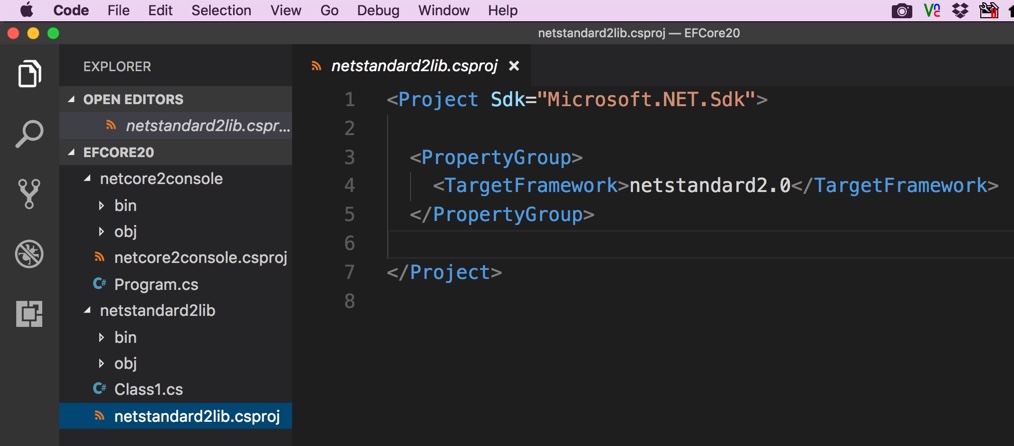
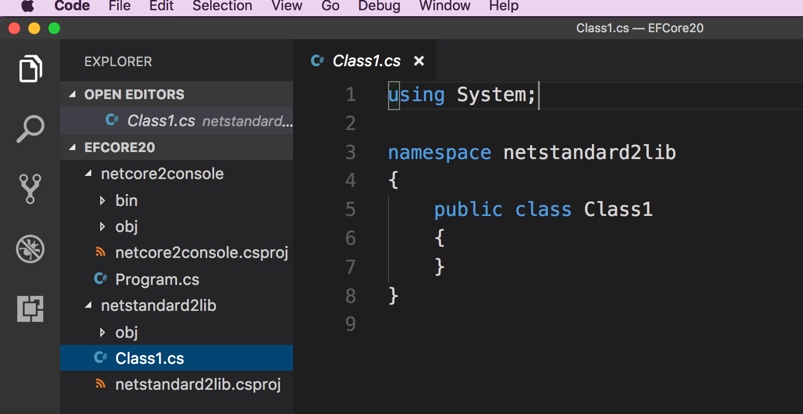
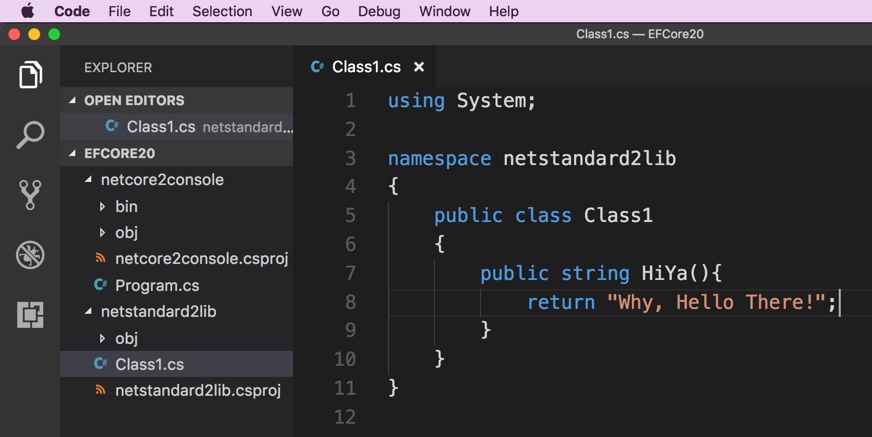
Now I’ll go into the csproj for the console app and give it a project reference to the library. The intellisense does not (yet?) help me with this and my memory sucks*, so I head to Nate McMaster’s handy project.json to csproj mind mapper aka Project.json to MSBuild conversion guide to remind myself the syntax of a project reference. I add this below the property group section in the console app’s csproj file
<ItemGroup> <ProjectReference Include="..\netstandard2lib\netstandard2lib.csproj"/> </ItemGroup>
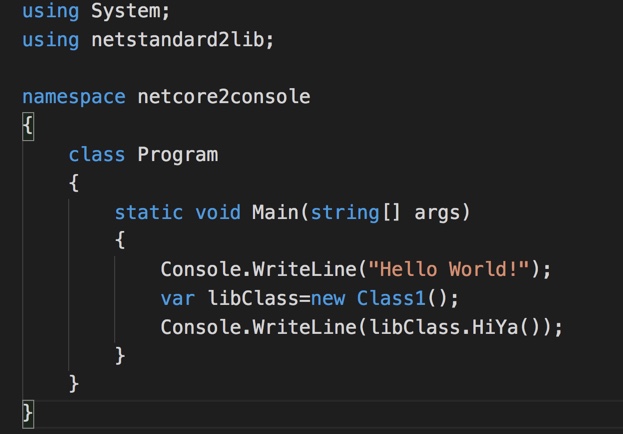
And then back out to my terminal window (though I can also do it inside VS Code’s built-in terminal). I dotnet build the library and then change to the console folder. Rebuild that and run it and …voila
➜ netcore2console dotnet run
Hello World!
Why, Hello There!
➜ netcore2console
So now I know that .NET Core 2 (preview from a nightly build) is running properly on my machine and that I can create and use a .NET Standard 2.0 library. That means I can go ahead and confidently start creating a .NET Standard 2.0 library to host some EF Core 2.0 logic.
I also repeated this entire thing on my Windows machine. Not only does that let me know I can use this version of .NET Core there, but I will also do some work from within Visual Studio with the .NET Core 2.0 preview.
*(duh, I should just make myself a snippet in VS Code!)
Sign up for my newsletter so you don't miss my conference & Pluralsight course announcements!
Julie,
Here we need a space between ProjectReference and Include –> ProjectReference Include
oh! thanks!! 🙂
Hi Julie, thank you for sharing your post showing us the newest .dot net core preview 2, please see my latest project in .dotnet core preview 2 called DDD Anemic Monolithic N-LayeredArchitecture with .Net Core Preview 2 https://github.com/cesarcastrocuba/nlayerappv3, its the newest version of N-LayeredArchitecture created by César de la Torre. If you want to collaborate/improve this project stay in touch with me in [email protected].